How to Build High-Performance Web Applications with .NET Framework
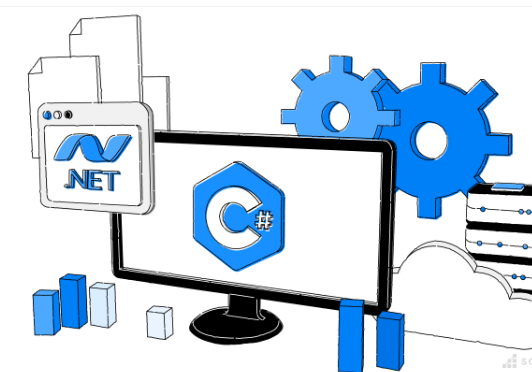
In today’s fast-paced digital world, users expect web applications to be fast, responsive, and efficient. Building a high-performance web application is essential for ensuring a positive user experience, reducing bounce rates, and improving search engine rankings. The .NET Framework, developed by Microsoft, provides a powerful environment for creating scalable and high-performance web applications.
This guide will walk you through the best practices, tools, and techniques for leveraging the .NET Framework to build web applications that are optimized for performance.
1. Introduction to the .NET Framework
The .NET Framework is a versatile software development platform developed by Microsoft, designed to support the creation of desktop, mobile, and web applications. Its comprehensive set of tools, libraries, and runtime environments makes it an excellent choice for building scalable, high-performance web applications.
The .NET Framework includes ASP.NET, which is specifically designed for web development, enabling developers to build dynamic, data-driven websites and applications efficiently.
See also: Top Features of .NET 7: What Developers Need to Know in 2024
2. Key Benefits of Using .NET for Web Applications
- High Performance: The .NET Framework is optimized for speed and reliability, making it ideal for performance-critical web applications.
- Scalability: Easily scale your applications as your user base grows.
- Robust Security: Built-in security features such as authentication, authorization, and data encryption.
- Cross-Platform Capabilities: With the introduction of .NET Core and .NET 7, web apps can run on Windows, Linux, and macOS.
- Rich Development Ecosystem: Comprehensive libraries, development tools, and strong community support.
3. Setting Up Your Development Environment
Before you start building your web application, ensure that your development environment is properly set up:
- Download and Install Visual Studio: The recommended IDE for .NET development is Visual Studio.
- Install the .NET SDK: Make sure you have the latest version of the .NET Framework installed.
- Set Up a Version Control System: Use GitHub or Bitbucket for source control to manage your code efficiently.
4. Best Practices for Building High-Performance Web Apps
To build a high-performance web application, it’s crucial to adopt best practices that optimize speed, scalability, and resource efficiency.
Optimizing Application Architecture
Designing a scalable and modular application architecture is key to ensuring high performance. Consider using the MVC (Model-View-Controller) pattern, which separates concerns and makes your codebase more manageable and efficient.
- Use Dependency Injection: Improves code modularity and testability.
- Implement Microservices Architecture: Break down large applications into smaller, independent services.
Efficient Caching Strategies
Caching is essential for improving the speed and efficiency of your web application:
- Output Caching: Store the results of expensive operations (like database queries) to reduce load times.
- Distributed Caching: Use tools like Redis or Memcached for scalable caching solutions.
Using Asynchronous Programming
Asynchronous programming can significantly improve the responsiveness of your web application, especially for I/O-bound tasks.
- Use async/await keywords to make your code non-blocking.
- Implement Task Parallel Library (TPL) for efficient background processing.
Minimizing Database Bottlenecks
The database is often a major bottleneck in web applications. Optimize your database interactions to improve performance:
- Use Stored Procedures: They are faster than ad-hoc queries.
- Implement Indexing: Improves query performance.
- Use Entity Framework: Optimize with AsNoTracking() for read-only queries to reduce overhead.
5. Leveraging ASP.NET for High-Performance Web Development
ASP.NET is the backbone of .NET web development, offering a robust environment for building high-performance web applications.
- ASP.NET Core: The latest version is cross-platform, lightweight, and optimized for performance.
- Razor Pages: Use Razor syntax for efficient and fast rendering of HTML pages.
- Middleware: Customize the request pipeline to optimize performance.
6. Using .NET Profilers and Performance Tools
To optimize the performance of your web application, it’s important to monitor and profile your code.
- Visual Studio Profiler: Provides detailed performance reports.
- dotTrace: A tool for profiling .NET applications to detect bottlenecks.
- Application Insights: A cloud-based service that provides real-time monitoring and diagnostics.
7. Enhancing Front-End Performance
The user experience is greatly affected by front-end performance. Consider the following tips:
Reducing Page Load Time
- Minimize HTTP Requests: Combine CSS and JavaScript files.
- Compress Assets: Use Gzip or Brotli compression for faster delivery.
- Use Content Delivery Networks (CDNs): Serve static content like images, CSS, and JavaScript files from CDNs.
Optimizing JavaScript and CSS
- Minify JavaScript and CSS: Remove unnecessary characters to reduce file size.
- Lazy Loading: Load images and scripts only when they are needed.
8. Security Considerations for Web Applications
Security is critical when developing web applications, especially those handling sensitive data.
- Authentication and Authorization: Use ASP.NET Identity or OAuth for secure user authentication.
- Data Encryption: Encrypt sensitive data using HTTPS and SSL.
- Prevent SQL Injection: Use parameterized queries or ORM tools like Entity Framework.
- Cross-Site Scripting (XSS) Protection: Sanitize user inputs to prevent malicious code execution.
9. Scaling Your Web Application for Growth
To handle increased traffic and data loads, consider implementing the following scalability strategies:
- Load Balancing: Distribute incoming traffic across multiple servers.
- Database Sharding: Split your database into smaller, more manageable parts.
- Horizontal Scaling: Add more servers to your infrastructure as your application grows.
10. Frequently Asked Questions (FAQs)
Q1. What is the difference between .NET Framework and .NET Core?
.NET Framework is primarily for Windows, while .NET Core (now unified as .NET 7) is cross-platform, open-source, and optimized for modern development.
Q2. How can I improve the performance of my ASP.NET application?
Use caching, asynchronous programming, and optimize database queries to improve performance.
Q3. Is it possible to deploy .NET applications on Linux?
Yes, with .NET Core and .NET 7, you can deploy .NET applications on Linux and macOS, in addition to Windows.
Q4. What are the best tools for profiling .NET applications?
Tools like Visual Studio Profiler, dotTrace, and Application Insights are excellent for monitoring performance.
Q5. How do I secure my .NET web application?
Implement HTTPS, use ASP.NET Identity for authentication, and sanitize user inputs to prevent XSS and SQL injection.
Conclusion
Building high-performance web applications with the .NET Framework requires a strategic approach, from optimizing backend architecture to enhancing front-end performance. By following best practices, leveraging modern tools, and focusing on scalability, you can create robust and efficient applications that meet the demands of today’s users.
The .NET ecosystem continues to evolve, offering powerful tools for developers to build high-quality applications. Whether you’re a beginner or an experienced developer, mastering .NET will empower you to deliver web applications that are fast, scalable, and secure.